Recipe CV Assistant with LLM API
As a demonstration of how to utilize Go4Lage, I present my entry to the Google Gemini AI Challenge. The objective was to create a web application leveraging the Gemini AI API. This challenge provided an opportunity to test Go4Lage, resulting in a functional backend with minimal effort, all sparked by a spontaneous decision after noticing the challenge on LinkedIn.
Gemini CV Features
Gemini CV performs two main functions:
- Generates a new CV for you.
- Optimizes the CV for maximum salary potential.
Gemini CV includes the following features:
- Allows users to upload their CV or draft in PDF or Text format.
- Analyzes the performance of the current CV.
- Generates an improved version of the CV.
- Compares the results and allows the user to choose the best version.
- Users can repeat the process iteratively, making manual adjustments in between.
This process provides users with an optimized CV, saving time and effort, and potentially increasing their salary by 5-10%.
Project Insights
This project served as a testing ground for the initial design of Go4Lage, helping to fix minor bugs and address unforeseen issues. While initially intended as a test, the project turned out to be both enjoyable and rewarding.
The development process was swift and straightforward, with minimal attention needed for bug fixes.
The result is a performant application with minimal vendor lock-in, and a codebase of roughly 1,000 lines in the backend (excluding prompts, of course).
Escaping vendor lock-in
The only significant dependency is the Gemini AI API, encapsulated in a custom wrapper that allows interchangeability with other LLM APIs, including free options like Ollama. While this might not align with Google's expectations, a development challenge must be open to outcomes that question the value of a specific business model. This approach is not only easier but also avoids many potential bugs.
func callGemini(instruction, prompt string, temp float32) (string, error) {
ctx, cancel := context.WithTimeout(context.Background(), time.Duration(Options.Scantimeouts)*time.Second)
defer cancel()
resultChan := make(chan string)
errChan := make(chan error)
client, err := genai.NewClient(ctx, option.WithAPIKey(Options.Key))
if err != nil {
return "", fmt.Errorf("failed to create client: %v", err)
}
defer client.Close()
model := client.GenerativeModel("gemini-1.5-flash")
model.SetTemperature(temp)
model.SystemInstruction = &genai.Content{
Parts: []genai.Part{genai.Text(instruction)},
}
go func() {
resp, err := model.GenerateContent(ctx, genai.Text(prompt))
if err != nil {
errChan <- fmt.Errorf("failed to generate content: %v", err)
return
}
if len(resp.Candidates) == 0 || len(resp.Candidates[0].Content.Parts) == 0 {
errChan <- errors.New("no content generated")
return
}
text, ok := resp.Candidates[0].Content.Parts[0].(genai.Text)
if !ok {
errChan <- errors.New("unexpected content type in response")
return
}
resultChan <- string(text)
}()
select {
case <-ctx.Done():
return "", errors.New("operation timed out")
case err := <-errChan:
return "", err
case result := <-resultChan:
return result, nil
}
}
Taking a Promise-like approach that leverages Go's native concurrency features, this wrapper elegantly manages LLM API communication through channels and timeouts - essential patterns for production-grade applications. By treating the LLM API as a simple text-in-text-out service with Promise-style asynchronous handling, we create a clean abstraction that's both powerful and portable.
The beauty lies in its ability to handle complex async operations with proper error boundaries and timeouts, while maintaining the flexibility to adapt to any AI provider's API with minimal changes.
Watch the Video
The competition required a video submission on YouTube. As a developer and not a video creator, the result may not be a masterpiece, but it gets the job done. Watch GeminiCV on YouTube.
Test GeminiCV Locally
Follow the steps below to run the app locally on your machine. Note that you will need your own Gemini API key for it to work:
#!/bin/bash
git clone https://github.com/Karl1b/go4lage.git
cd go4lage
git checkout geminicv
cp dockerenv.env .env
# Adjust the .env file as needed
docker-compose build
docker-compose up -d
docker exec -it geminicv_app /app/go4lage createsuperuser
You can now go to the URL /admin to use Go4Lage's admin dashboard.
Screenshots
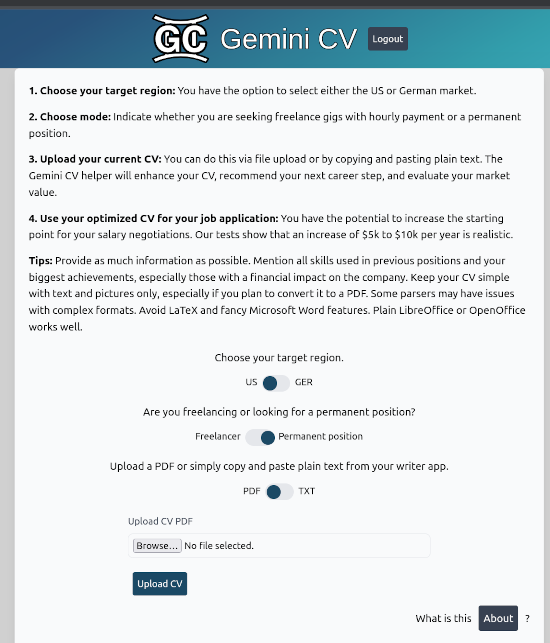
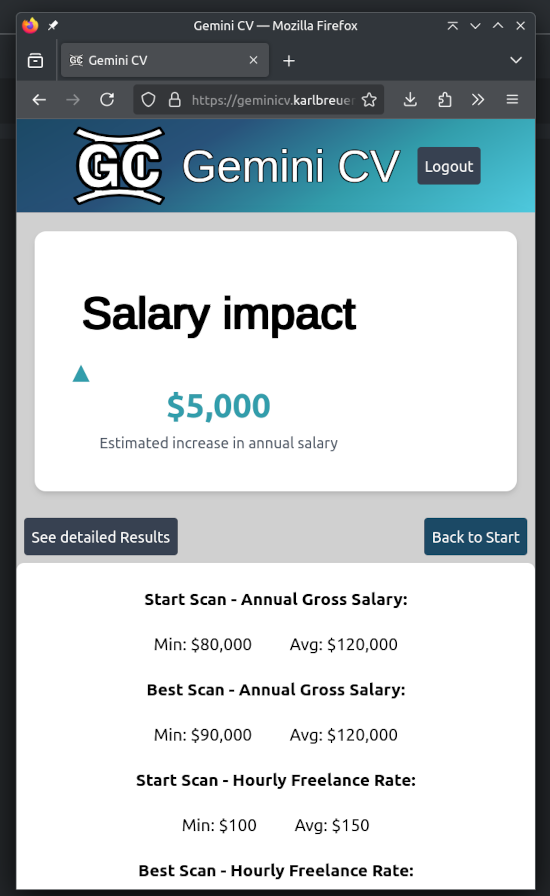
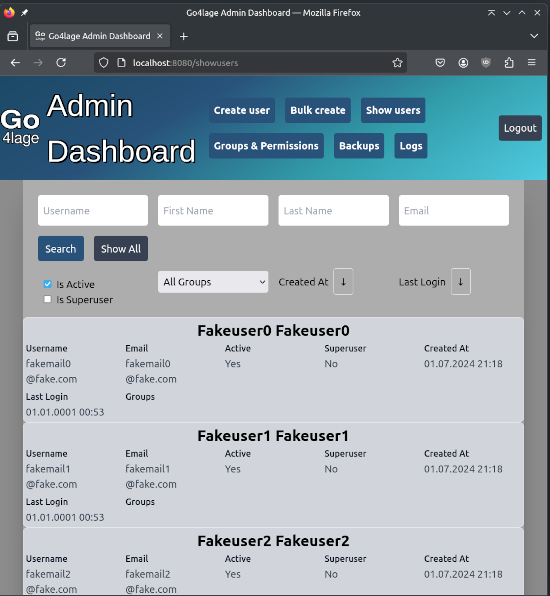
Steps to Create the Backend
This is a brief walkthrough. Simply git checkout geminicv to see the code.
SQL Database / Model
- Create your SQL schema at ./pkg/sql/schema/: Use native SQL to define it. This should be well-defined before going into production. Do not modify the base structures; instead, extend them as needed.
- Create your SQL queries at ./pkg/sql/queries/: Use native SQL to define them. LLMs can assist with more complex SQL queries.
- Automatically generate the Go queries: Run
sqlc generate
. This will provide all the necessary Go code with autocomplete support.
In general: Avoid using a different database like SQLite for development and production. Instead, use Postgres as indicated in the setup. ./go4lage rungoose up
and ./go4lage rungoose down
will be your go-to commands. This approach is faster than deleting and recreating your SQLite database.
Endpoints
This is the logic layer of the application.
- Create a specific app folder for your package: In this case, it is
./pkg/geminicv
. - Create your package Go files: Use two files, one for the prompts and one for the endpoint. Access the user via the context:
user, ok := r.Context().Value(utils.UserKey{}).(db.User)
.
Register API Endpoints
Register your endpoints in the url.go
file.
- Initialize your app struct: This gives you access to the SQL queries and .env options.
- Route your endpoints: Use the appropriate authentication middleware with permissions and groups if needed.
.env Configuration
For this app, three additional settings are required:
- API Key:
GEMINIKEY=y0urS3cr3tKeyGo3sheReDon0tShare
- Limit on runs per user to prevent abuse:
CVRUNS_PER_USER=20
- Timeout for the Gemini LLM API response:
SCAN_TIMEOUT_SECONDS=211
Frontend Integration
Go4Lage is not specific to React, and it's not necessary to let Go4Lage serve the frontend. In this example, I used the ./root
folder as the build destination for my React Vite builder. This approach creates an all-in-one package, but it is optional. You can point your frontend endpoints to the backend and be ready to go.
The React frontend is also provided as an example in this repository.